Building Scalable Apps with Redis and Node.js: A Comprehensive Guide

Redis is an in-memory data structure store that is often used as a cache or a database. It is known for its speed and scalability, making it a great choice for building applications that need to handle large amounts of data.
Node.js is a JavaScript runtime that is often used for building web applications. It is known for its event-driven architecture and its ability to handle a large number of concurrent connections.
Together, Redis and Node.js make a powerful combination for building scalable applications. In this guide, we will cover everything you need to know to get started with Redis and Node.js, including:
4.1 out of 5
Language | : | English |
File size | : | 8743 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 318 pages |
- Basic Redis concepts
- How to use Redis with Node.js
- Advanced Redis techniques
- Best practices for building scalable applications with Redis and Node.js
Redis is a key-value store that uses a hash table to store data. Each key is a string and each value can be a string, a list, a set, or a hash.
Redis supports a variety of operations, including:
- GET: Retrieve the value of a key
- SET: Set the value of a key
- DEL: Delete a key
- LPUSH: Push a value onto a list
- RPUSH: Pop a value from a list
- SADD: Add a value to a set
- SMEMBERS: Get all the members of a set
- HSET: Set a field in a hash
- HGET: Get the value of a field in a hash
- HDEL: Delete a field from a hash
For more information on Redis commands, please refer to the Redis documentation.
There are a number of Node.js modules that can be used to interact with Redis. In this guide, we will use the redis
module.
To install the redis
module, run the following command:
npm install redis
Once the module is installed, you can require it in your Node.js code:
const redis = require('redis');
To create a Redis client, use the createClient()
method:
const client = redis.createClient();
Once you have a Redis client, you can use it to perform Redis commands. For example, to set the value of a key, use the set()
method:
client.set('foo', 'bar');
To get the value of a key, use the get()
method:
client.get('foo', (err, reply) => { if (err) throw err; console.log(reply); });
<h2>Advanced Redis Techniques</h2> Redis supports a number of advanced techniques that can be used to improve the performance and scalability of your applications. These techniques include: * **Sharding:** Sharding is a technique that involves splitting a large Redis instance into multiple smaller instances. This can help to improve performance and scalability by distributing the load across multiple servers. * **Replication:** Replication is a technique that involves creating multiple copies of a Redis instance. This can help to improve availability and fault tolerance by ensuring that there is always a backup instance available in case of a failure. * **Clustering:** Clustering is a technique that involves connecting multiple Redis instances together to form a cluster. This can help to improve performance and scalability by distributing the load across multiple servers and by providing a single point of access to the cluster. For more information on advanced Redis techniques, please refer to the Redis documentation. <h2>Best Practices for Building Scalable Applications with Redis and Node.js</h2> When building scalable applications with Redis and Node.js, it is important to follow a number of best practices. These best practices include: * **Use Redis as a cache:** Redis is a great choice for caching data that is frequently accessed. This can help to improve the performance of your application by reducing the number of times that you need to access the database. * **Use Redis as a database:** Redis can also be used as a database for storing data that does not need to be stored in a relational database. This can help to improve the scalability of your application by reducing the load on your database server. * **Use Redis for message queuing:** Redis can also be used for message queuing. This can help to improve the performance and reliability of your application by decoupling the different components of your application. * **Configure Redis appropriately:** It is important to configure Redis appropriately to meet the needs of your application. This includes configuring the number of shards, replicas, and clusters that you need. By following these best practices, you can build scalable applications with Redis and Node.js that can handle a large amount of data and traffic. Redis is a powerful tool that can be used to build scalable and high-performance applications. By following the tips and tricks in this guide, you can</body></html>
4.1 out of 5
Language | : | English |
File size | : | 8743 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 318 pages |
Do you want to contribute by writing guest posts on this blog?
Please contact us and send us a resume of previous articles that you have written.
Book
Novel
Page
Chapter
Text
Story
Genre
Reader
Library
Paperback
E-book
Magazine
Newspaper
Paragraph
Sentence
Bookmark
Shelf
Glossary
Bibliography
Foreword
Preface
Synopsis
Annotation
Footnote
Manuscript
Scroll
Codex
Tome
Bestseller
Classics
Library card
Narrative
Biography
Autobiography
Memoir
Reference
Encyclopedia
Joanne Gray
Chongbin Zhao
Pierre Moniz Barreto
Thomas Cleary
Charles C Bates
James Kakalios
Charles Sumner
Helen K Gediman
Cathy Camper
Jo Anne Preston
William Walker Atkinson
Cheryl R Shrock
Matt Rosen
Chris Robertson
Charles K Coe
Joost Abraham Maurits Meerloo
Sharanabasava V Ganachari
Chaim Bezalel
Christa Mackinnon
Thomas D Wickens
Light bulbAdvertise smarter! Our strategic ad space ensures maximum exposure. Reserve your spot today!
- Lawrence BellFollow ·15.7k
- Brady MitchellFollow ·2.4k
- Pablo NerudaFollow ·3.2k
- Joseph HellerFollow ·12.6k
- Ian PowellFollow ·5.5k
- Ralph TurnerFollow ·11.8k
- Luke BlairFollow ·8.6k
- Matthew WardFollow ·6.4k
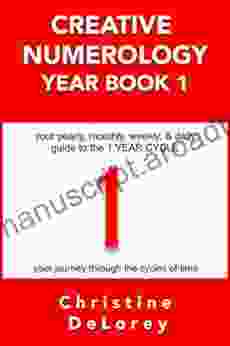

Your Yearly Monthly Weekly Daily Guide To The Year Cycle:...
As we navigate the ever-changing currents...
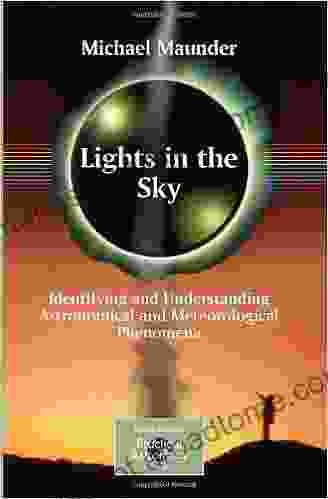

Identifying and Understanding Astronomical and...
Prepare to embark on an extraordinary...
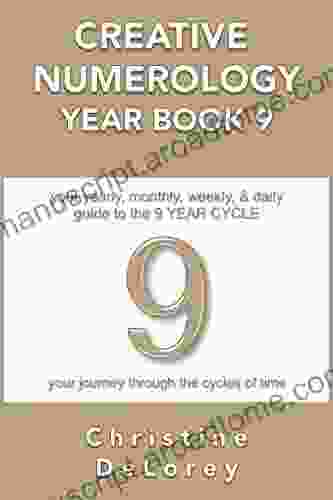

Your Yearly Monthly Weekly Daily Guide to the Year Cycle:...
Welcome to "Your Yearly Monthly Weekly Daily...
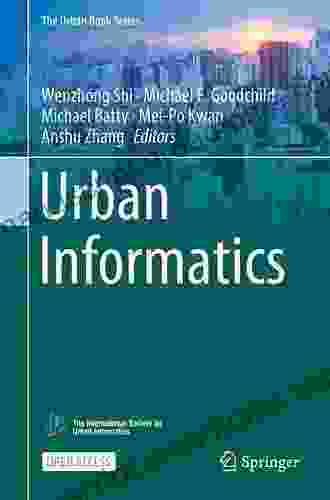

Urban Informatics: Unlocking the Secrets of Smart Cities...
An In-Depth Exploration of Urban...
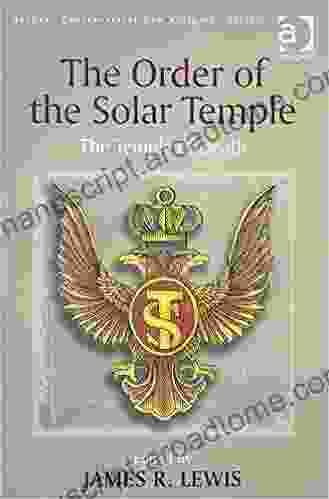

Unveil the Secrets of the Order of the Solar Temple: A...
In the realm of secret...
4.1 out of 5
Language | : | English |
File size | : | 8743 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 318 pages |